API Usage (Part 2)
OpenSource
- No personal information stored on file.
- Payments made exclusively in BTC.
- OpenSource is not responsible or liable if you are charged criminally.
In this part of the project we are going to work on what happens when the user clicks the search button. It is basically an API call that goes through instantly, but we are going to make it look like it is reaching out and searching for developers in the area.
The HTML and CSS will change the landing page to a searching page with a rotating icon inside of it. We will add a few effects, like random time to search, to mimic what a real-life GPS search would feel like.
The JavaScript will add some changing text to the search and implement a few random Math methods to give implement some random timing.
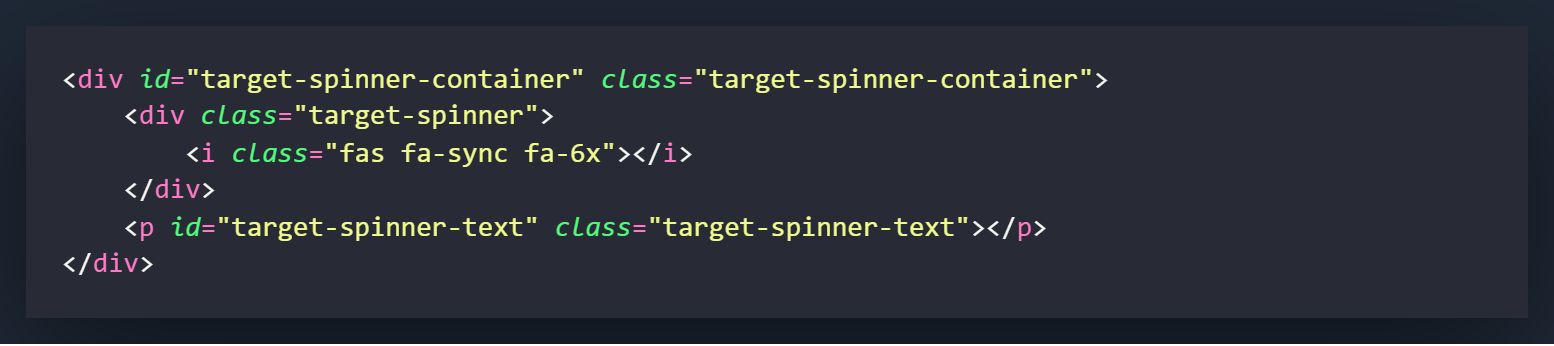
The above code is what we will be adding to the existing HTML code. If you look at the code snippet below this section, the area marked with the comment SPINNER CODE START is where you want to start writing the above HTML code.
The .target-spinner-container will be what houses the spinner content. It is basically functioning how the landing page was in the first section of this tutorial. This is what will be replacing the landing page when the user clicks the search button.
Inside the .target-spinner-container div, we will have two separate sections. One will contain the spinning icon for the search and the second will contain the text that will change as the search takes place.
The spinner I used from font-awesome can also be replaced with any loading spinner you would like. In this tutorial it will be spinning clockwise.
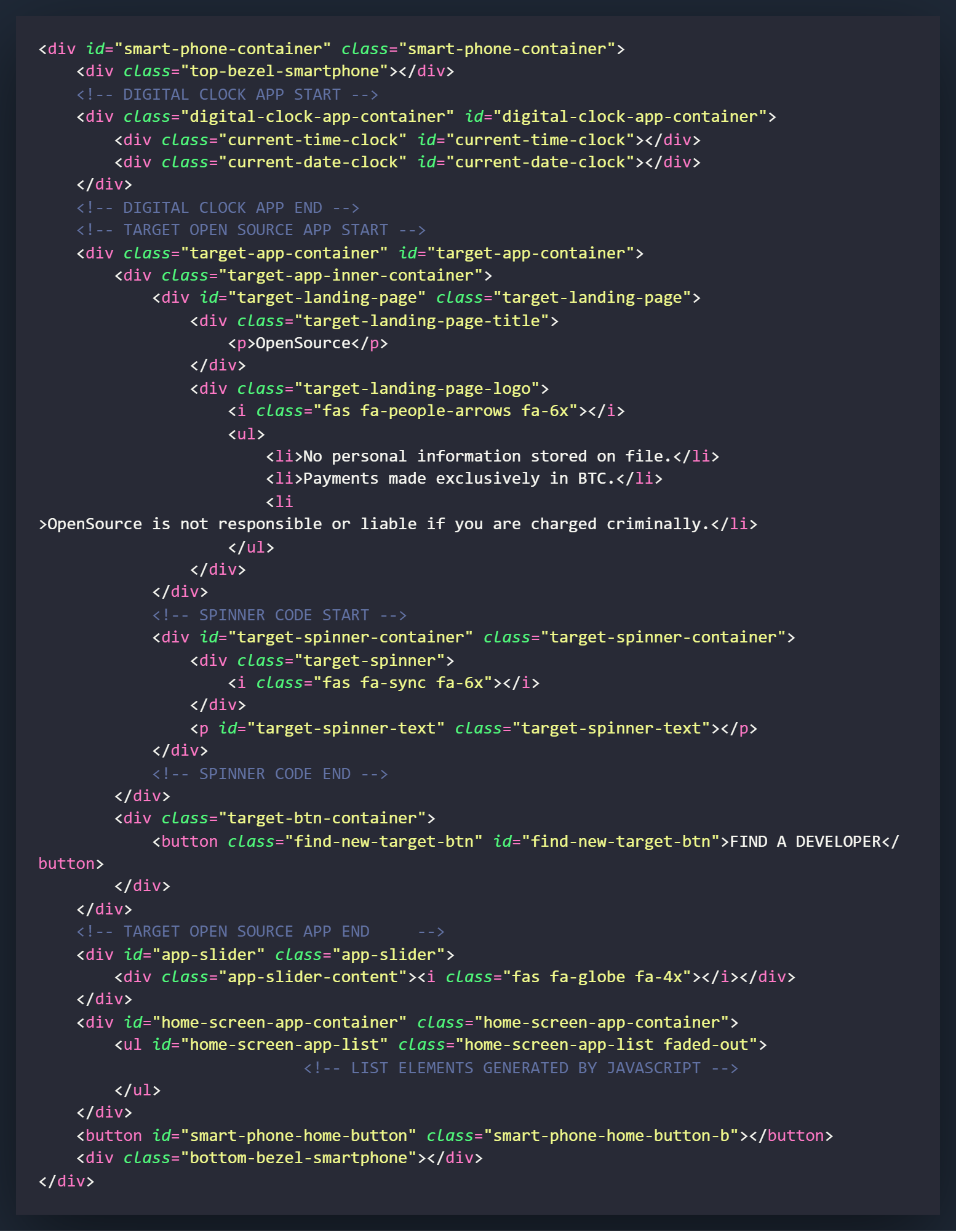
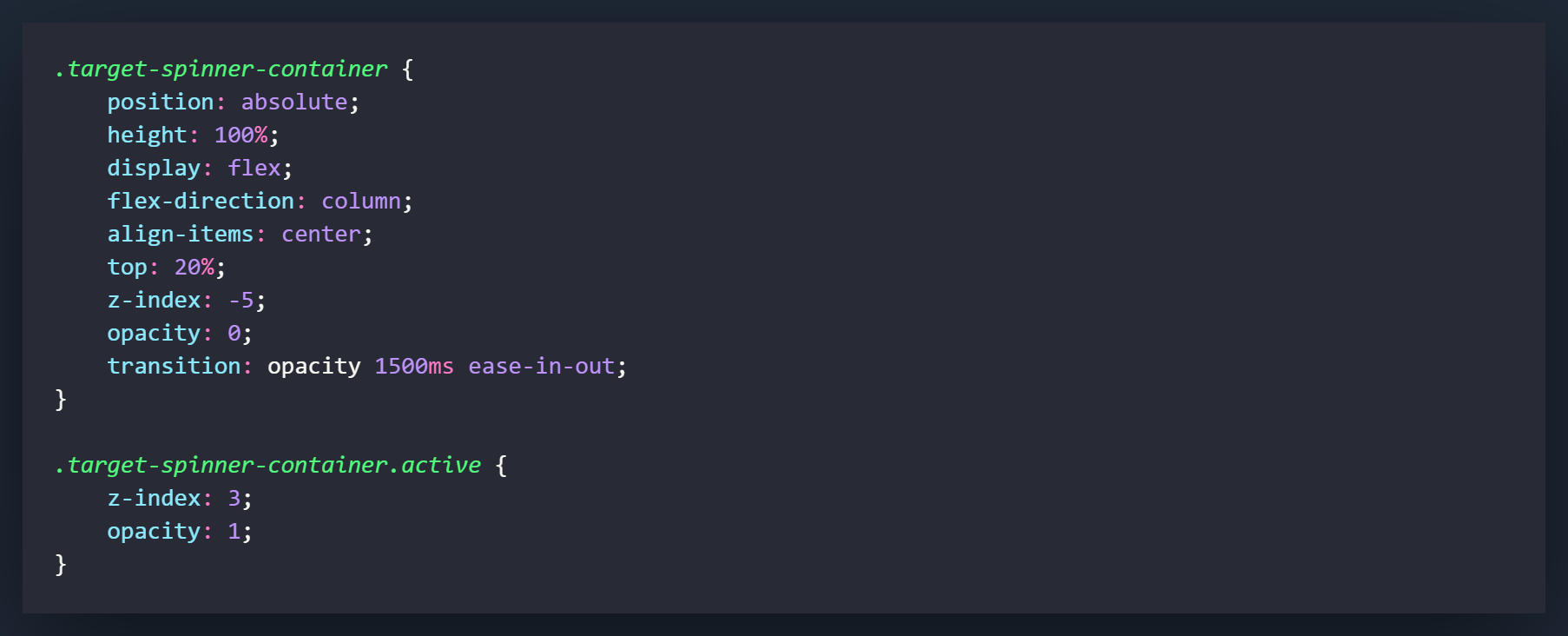
First we will style the .target-spinner-container. We are going to make this div fade in when it is called, so instead of sliding in from another position, we will have it sitting below the screen on the z-axis. Many of the settings on here will relate to how the .target-spinner-container appears on the screen but the z-index setting and the opacity setting will stop it from appearing when it is not needed. The transition effect on the opacity will control how the div appears and in this case it will fade in by gradually bringing the opacity from 0 to 1.
.target-spinner-container.active settings will come into effect when the .active class is added. This will cause the div to fade in and appear. The z-index setting of 3 will allow the div to rise above everything else and appear on the screen.
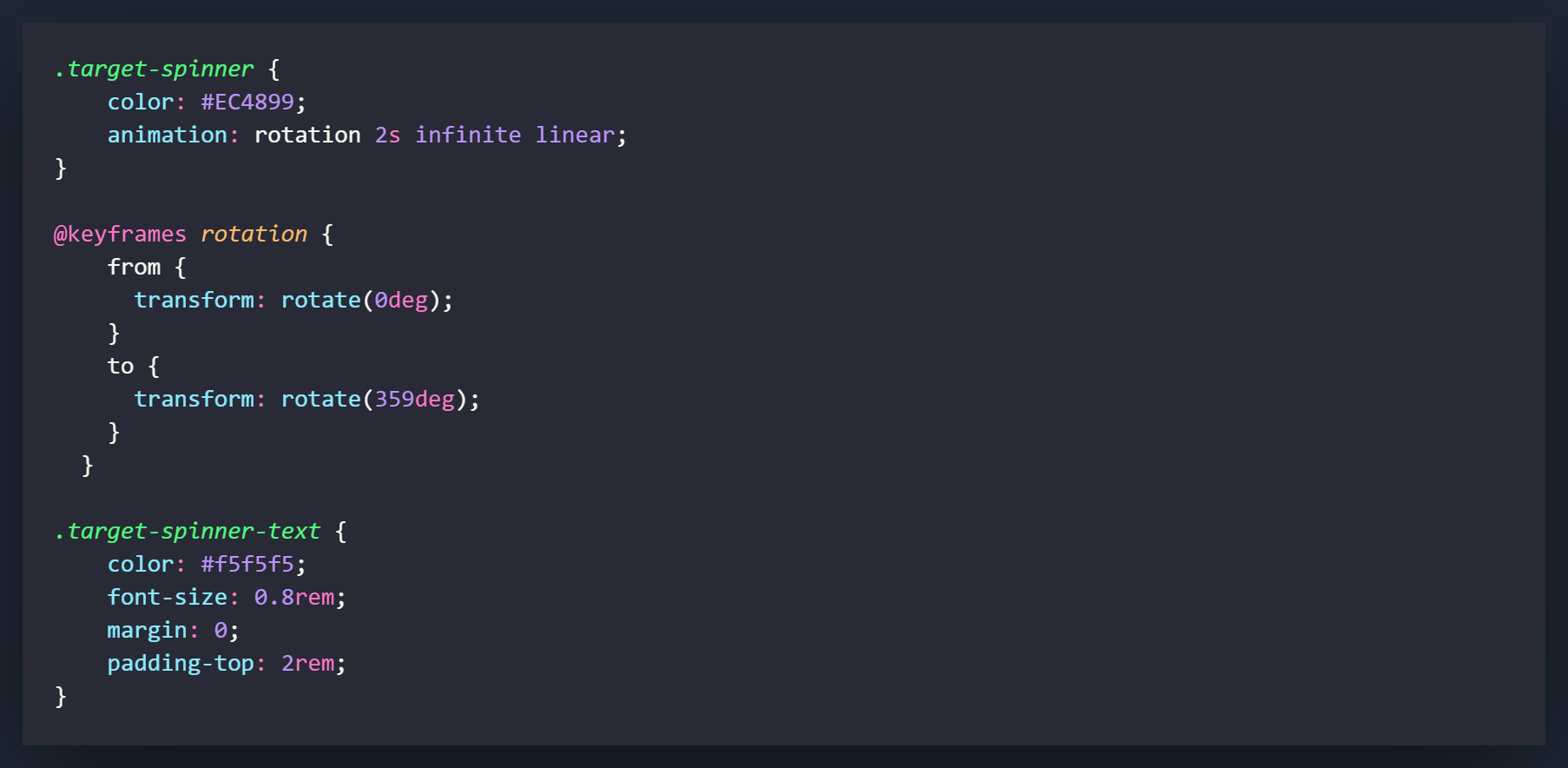
.target-spinner is the div that will be holding the icon that relates to the search. The animation setting sets what we will be doing and that will be set in the next section when we set the keyframes. Keyframes allow us to tweak certain things about animations and in this case we will be making the rotation animation rotate from 0 degrees to 359 degrees in a clockwise motion.
The .target-spinner-text class is just used for styling the words that appear below the spinning icon.
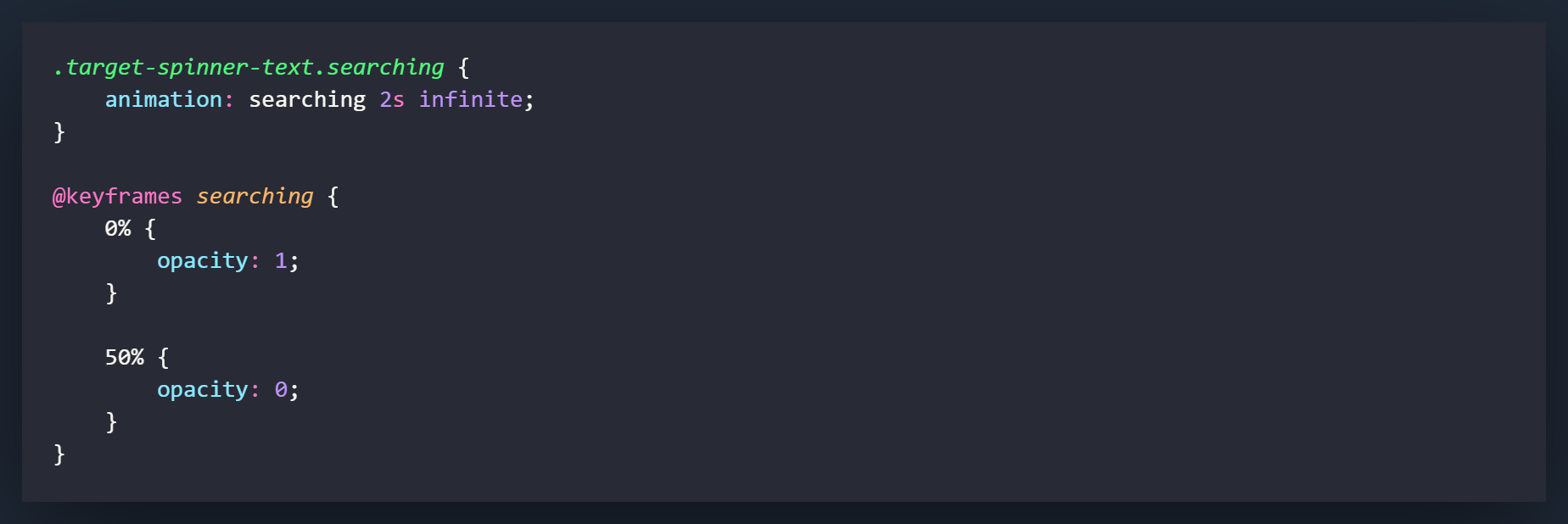
To add a neat loading trick to the words below the icon, we will set it to fade in and out as it conducts its search. Like the rotation, we will accomplish this in much the same way. When the class of .searching is added to .target-spinner-text it will activate the searching animation that will cause the words to start at an opacity of 1 and fade out to 0 over 2 seconds. This will continue to repeat until our search concludes.
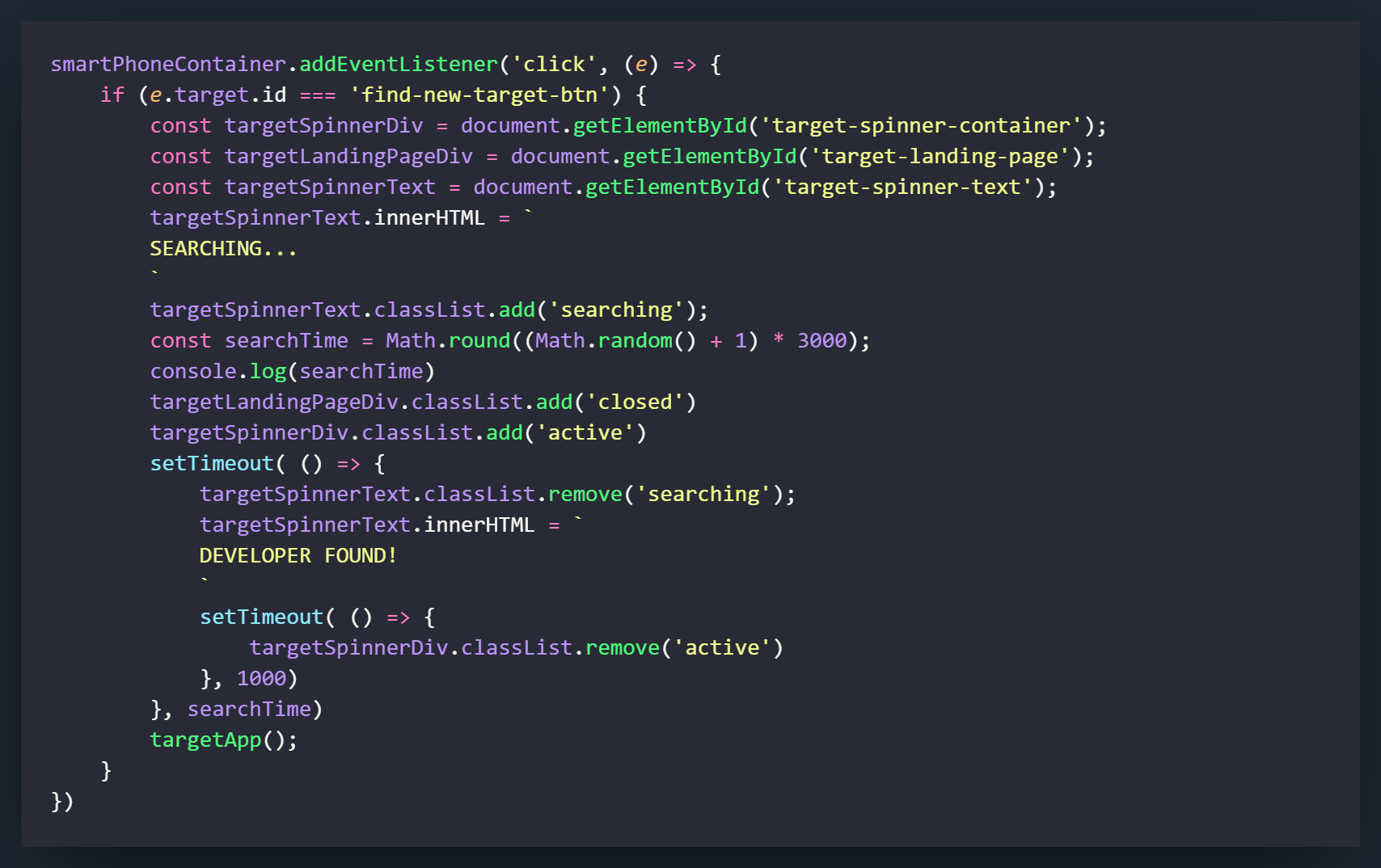
Now we are going to build out the functionality for the .find-new-target-btn. Clicking this button will cause the search to start and make an API call to get a random person. We are not going to deal with API quite yet but we will set everything up to appear like gears are turning as this call is made. It's similar to an optical illusion and is something that is used all over the modern web. A loading animation is used just in case the data takes a little while longer to fetch.
The very first thing we will add is an eventListener on the smartPhoneContainer. We will pass a click event into the function (e) that will call an IF statement that will look for an element that has the target ID of find-new-target-button. When that element is clicked on it will lead into 3 variables being created - targetSpinnerDiv, targetLandingPageDiv, and targetSpinnerText.
The first variable we will change will be the innerHTML of the targetSpinnerText variable. We will add the text of SEARCHING to the innerHTML and right after that the class of searching to the element. If you remember in the CSS, this will cause the element to fade in and out to simulate that something is being searched.
Now we are going to create a little bit of an optical illusion. Generally an API call will be instant and it would never be necessary to fake the illusion of a search. Honestly though, that kind of takes the fun out of things. What we will do here is create a random variable, as signified by the variable searchTime. This searchTime variable is going to give us a random number between 3000 and 6000 milliseconds. I have added a console.log(searchTime) so you can see it for yourself.
The next two lines are actually what will make the landing page disappear and the targetSpinnerDiv appear. What you will find yourself doing a lot of the time with Vanilla JavaScript is finding ways to buffer in your function calls that doesn't interfere with how things appear. This means making calls at the right time so they can function before divs can change state and move around. It all happens in milliseconds and the outcome is still the same but it can make a difference with how things appear.
Now we are going to use a setTimeout that will utilize our searchTime variable, which you can see at the very bottom of the code block in the position that a time in milliseconds would generally be used.
We will be using two setTimeout methods here, the second will go into effect when the first one completes. The first one will end when the searchTime variable in milliseconds runs through. When that completes, the innerHTML that showed SEARCHING will now show DEVELOPER FOUND and the fading out pulse will stop.
There are 2 things left that will run in this function. The first will be removing the class of .active from the targetSpinnerDiv causing it to fade out, and the second will be calling the targetApp( ) function.
In our next section we will create the function that makes the API call and fills out the DOM with info from it.